What is Hackvertor (and why should I care)?
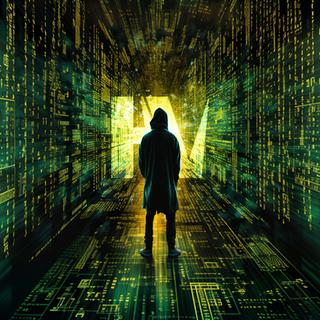
What’s Hackvertor and why should I care?
Years ago, Gareth Heyes created a Burp Suite (Burp) extension called Hackvertor. It’s an extension with a lot of features, but at its core, it allows you to add tags around sections of an HTTP request to dynamically encode, decode, encrypt, decrypt, convert, and hash, along with a bunch of other functions. It’s not a pro extension, so it works on both the pro and community versions of Burp, and it allows tags in the major sections of Burp, including Repeater and Intruder.
So why am I bringing up an extension that’s been around for a while? Well, when mentioning its capabilities to colleagues, it didn’t seem well known, and I find it very useful in modern web assessments to increase productivity. So, I thought I’d share some use cases I ran across recently.
Usage
Directions on how to install the extension and add tags to requests are documented in the extension’s GitHub, but the basics on how to use the extension are:
- Highlight a section in a Repeater request
- Right-click on the highlighted section
- Select “Extensions” from the right-click menu
- Select “Hackvertor”
Choose a function.
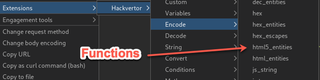
Tags will get set around your selected text.

If you want to see what a sent request looks like after the tags have been processed, view the logger tab in Burp and sort the view table in descending order.

The request will contain whatever value was returned by the Hackvertor function used. In the example above, we are URL encoding a request parameter.
Intruder
A useful feature of the extension is that it can be used in other tabs in Burp, including Intruder.
Let’s take an example of modifying an authorization token. A JSON Web Token (JWT) is typically broken up into three (3) parts, and each part is Base64 encoded. In some cases, applications do not verify the signature of a JWT, so an attacker can modify the body of the token to access another user’s data. To modify a JWT token, decode the body of the token using built-in Burp features like the convert function.
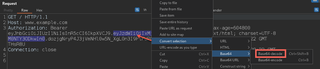
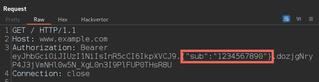
Then, use Hackvertor to dynamically encode the body of the token.
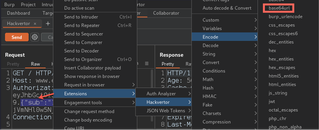
Tags will then be set around the token’s body.
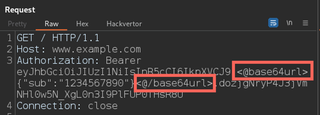
With the token body now decoded and surrounded by a Hackvertor tag, we can now brute force a section of the token’s body. To do this, we select a section of the token we want to brute force and send the request to Intruder.
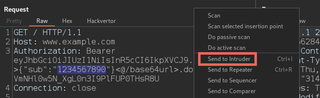
TIP: If you select a section of a Repeater request before you send it to Intruder, it will set a position at that location in Intruder. This ensures you don’t have to select a section manually.

If we want to loop though parameters in the token body, we could use a simple list of numbers and initiate an Intruder attack.
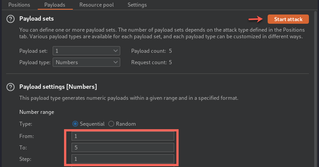
After our attack, if we want to verify that requests are being sent in the correct format, we can go to the logger tab and view our requests.
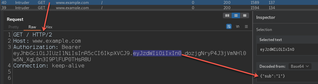
In the logger tab, we can see Hackvertor re-encodes the token body before sending, making it easier to brute force encoded values.
After the Intruder attack is finished, we can look through the results for any responses that seem to have returned valid data. Typically, you can use the response length or response code to identify interesting responses. If a response length is greater than other responses or the response code is the same as the original unmodified request, these things should be reviewed.
Custom Variables
Another interesting feature of Hackvertor is the ability to set custom variables. You can set and get variables by defining your own tags.
To set a variable:
<@set_this>this<@/set_this>
To return a variable:
<@get_this/>
This can be useful for requests that validate data using signature values.
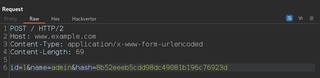
The request above requires an MD5 hash value of the body parameters to be sent with each POST request. Instead of having to recalculate the hash manually every time we want to change a body parameter, we can have Hackvertor calculate it for us.
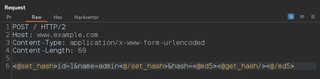
To calculate the hash, we set a Hackvertor variable around what we want to hash (i.e., the first two (2) body parameters). In the "hash" parameter, we get the value of our variable and surround that with an MD5 Hackvertor tag.
Now if we needed to change the value of "id" or "name" in our POST body, the "hash" will be calculated for us.
Global Variables
The file menu for Hackvertor that’s located at the top of Burp allows several different options, including the ability to set global variables. Once a variable is added you can return its value by using the get variable tag.
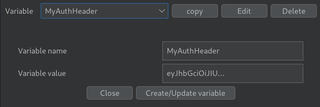
This can be useful if you have a few Repeater requests that have the same value such as a checkout process with multiple steps. To use a global variable, replace a section of the request such as the Authorization header with a get variable tag.
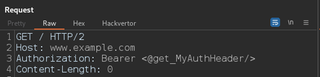
Now every time your authentication expires, you can just update the global variable with a valid JWT and all the requests you have saved in Repeater are now authenticated again.
Encryption
Another interesting feature of Hackvertor is that you can encrypt and decrypt values. On a recent engagement, we discovered an encryption key and IV value in a JavaScript file. When the application sent requests, it would encrypt URL parameters for an extra layer of security.
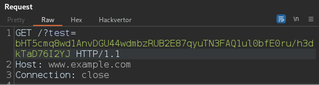
Using Hackvertor’s standalone tab, we can pass the URL parameter to a decrypt function with the encryption key and IV that we discovered. Hackvertor has its own tab similar to Repeater or Intruder where you can add tags and view the output. This works well on tags where you are unsure what the output will be like when decrypting.
Note: This tab is different than the tab in the Repeater window.

In the decrypted text, we see report numbers. A common thing we would want to try is to see if we can return other valid reports by brute forcing report numbers. To easily modify the report number, we can add a Hackvertor encrypt tag around our decrypted JSON and set the Hackvertor tag as the URL parameter value.
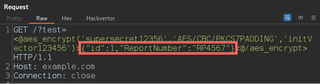
Then we can send the request to Intruder with a position set on the report number.

This allows us to quickly find other valid reports that would have otherwise taken a while to manually find. Hackvertor encryption uses the same algorithm names that are used in Java’s cryptography libraries so if you need to use any other algorithm names they can be found in common documentation for Java or Oracle.
Code Execution
The file menu for Hackvertor also has an option to "Allow code execution tags".
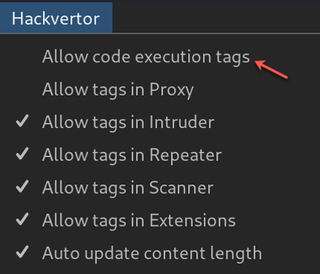
This allows you to run code dynamically. To view your code output, use the Hackvertor tab like we did when we were decrypting values.
To add a Hackvertor Python script to a request, you must have "Allow code execution tags" checked in the Hackvertor menu, and you must have the Python environment set up in Burp. This normally just requires you to download the latest Jython JAR file and link the location in Burp's extension settings. Many extensions require Jython, so you may already have it. If not, click the question mark in the extension settings to help you set it up.
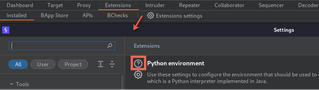
With everything set up, go to the Hackvertor tab, and select Languages > python.
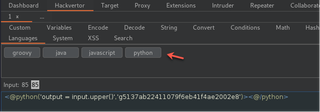
Hackvertor code tags add an execution key for security, the key must be included in any requests, or the code will not execute. When you add a Hackvertor Python tag, the key is automatically generated.
The Python tag takes two (2) values: the code and the execution key.
python('output=<ValueYouWantToDisplay>', 'execution key')
An example of where this can be useful is when you want to run repeated logic in a request. Recently, I came across some functionality in an application that allowed a database query.
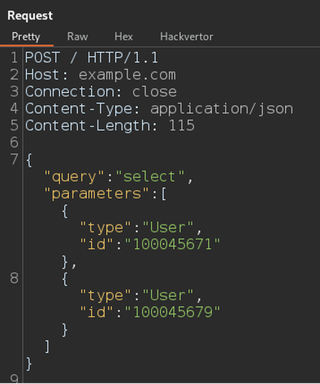
Not finding any common SQL injection (SQLi) vulnerabilities, I wanted to see if I could return other users. I could just send this to Intruder and increment one (1) of the user IDs, but this request is allowing multiple users to be selected in a single request, which is common in GraphQL queries. So instead of sending 1,000 requests to the server, we can add 1,000 users to the POST body of a single request.
Doing this manually is obviously not a good use of time, so typically I would write a script to generate a list of users. However, instead of doing this outside of Burp, I can do this quickly in a Hackvertor tag. As an example, we can create a numbered loop and modify the last number of the user ID.
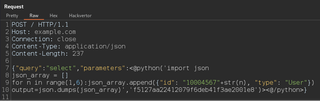
<@python('import json
json_array = []
for n in range(1,6):json_array.append({"id": "10004567"+str(n),"type":"User"})
output = json.dumps(json_array)', 'execution key')>
<@/python>
Python relies on spacing, and we can match the indentation in a Hackvertor tag with a new line. Now if we look at the request sent in the logger tab, we can see it created a JSON list of possible user IDs.
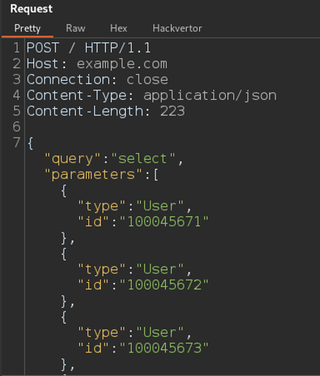
Obviously, you can use any code you would like and, in the past, accessing data from the file system has also proven helpful.
Summary
Manual testing is still one of the best ways to find impactful vulnerabilities, but that doesn’t mean you have to do everything manually. Anything that can save time can help cover more ground in an application, and the more functionality that is reviewed, the better the chance of finding a vulnerability. There are plenty more functions to use that we didn’t cover. Feel free to add the extension and play with it yourself.
Shoutout to Gareth for making the plugin and maintaining it all these years.